We have already used top-down programming to solve problems. In our exploration of bacterial genomes in Chapter 1, we wrote a functionย ReverseComplement()
ย to take the reverse complement of a DNA string by assuming that we had two simpler functionsย Reverse()
ย andย Complement()
.
ReverseComplement(text) x โ Reverse(text) y โ Complement(x) return y
We also applied top-down programming when we simulated many elections by assuming that we had already written a subroutineย SimulateElection()
ย that would handle simulating a single election.
SimulateMultipleElections(polls, electoralVotes, numTrials, marginOfError) winCount1 โ 0 winCount2 โ 0 tieCount โ 0 for numTrials total trials votes1,votes2 โ SimulateElection(polls, electoralVotes, marginOfError) if votes1 > votes2 winCount1 โ winCount1 + 1 else if collegeVotes2 > collegeVotes1 winCount2 โ winCount2 + 1 else (tie!) tieCount โ tieCount + 1 probability1 โ winCount1/numTrials probability2 โ winCount2/numTrials probabilityTie โ tieCount/numTrials return probability1, probability2, probabilityTie
The SimulateOneElection()
function in turn called an AddNoise()
subroutine, which is where the gritty details of the program were hiding in the form of (pseudo)random number generation, which required its own set of subroutine calls.
As we move toward larger programs with many functions, we can visualize how these functions are organized using a function hierarchy, or a network in which functions are connected to subroutines that they call by arrows. In a function hierarchy, the โrootโ function (i.e., the one at the top of the diagram that has no incoming arrows) solves the computational problem at hand. Function hierarchies are shown in the figure below for the functions SimulateMultipleElections()
and ReverseComplement()
.
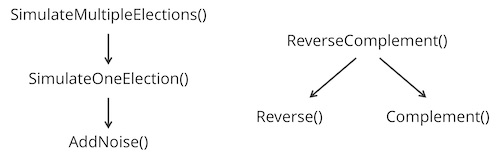
Exercise: Review the Game of Life Problem from the previous lesson. Plan the highest-level function in the hierarchy that will solve this problem. What subroutine(s) will be helpful?